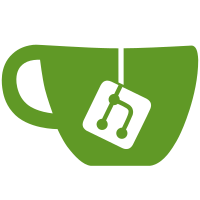
Updated tutorials to fix minor bugs and pathing issues. Also fixed crash bug on failure to create the audio object. Added msvc projects for all tutorials. Updated the listener class to be self contained and only handle stuff related to the OpenAL listener. It does NOT init OpenAL anymore, that has been moved to cAudioManager. Extended the listener class to support all settings that native OpenAL supports. Cleaned up cFileSource and fixed a crash bug on NULL file handle Fixed returning bad audio buffer chunk sizes from the cOggDecoder on errors in the ogg stream Seeking can now be down in fractions of a second now, changed the seconds field from int to float Fixed various odd formatting. Fixed potential crash bug in cMemorySource if memory could not be allocated. cMemorySource will no longer clear the buffer you give to it before filling it with data. This prevents an overwrite from happening in case of error but the user should provide a zeroed buffer to cMemorySource anyway for safety. Relative seeking is now supported by cOggDecoder.
92 lines
2.5 KiB
C++
92 lines
2.5 KiB
C++
#ifndef CAUDIO_H_INCLUDED
|
|
#define CAUDIO_H_INCLUDED
|
|
#include <string>
|
|
#include <iostream>
|
|
#include "AL/al.h"
|
|
#include "AL/alut.h"
|
|
#include <vector>
|
|
|
|
#define BUFFER_SIZE ( 1024 * 32 )
|
|
#include "../include/IAudio.h"
|
|
namespace cAudio
|
|
{
|
|
class cAudio : public IAudio
|
|
{
|
|
public:
|
|
//! play with defualts / the last set values
|
|
bool play();
|
|
//!plays the audio file 2d no distance.
|
|
void play2d(bool loop = false);
|
|
//!plays the audio file and sets it to 3d
|
|
void play3d(bool loop = false, float x = 0.0, float y = 0.0, float z = 0.0, float soundstr = 1.0);
|
|
//!allows us to set the position or reset the position
|
|
void setPosition(float posx,float posy,float posz);
|
|
//!allows you to set the audio objects velocity
|
|
void setVelocity(float velx,float vely,float velz);
|
|
//!allows us to set the direction the audio should play in / move
|
|
void setDirection(float dirx,float diry,float dirz);
|
|
//! Sets the audios pitch level
|
|
void setPitch(float pitch);
|
|
//!allows us to set and reset the sound strenght
|
|
void setStrength(float soundstrength);
|
|
//!Set the volume
|
|
void setVolume(float volume);
|
|
//!Set the doppler strength
|
|
void setDopplerStrength(float doop);
|
|
//!Set doppler velocity
|
|
void setDopplerVelocity(float doopx,float doopy,float doopz);
|
|
//!Seek the audio stream
|
|
void seek(float secs);
|
|
//!release the file handle
|
|
void release();
|
|
//!pauses the audio file
|
|
void pause();
|
|
//!controls altering of the looping to make it loop or not to.
|
|
void loop(bool loop);
|
|
//!stops the audio file from playing
|
|
void stop();
|
|
|
|
//!returns if playing
|
|
bool playback();
|
|
//!check if source is playing
|
|
bool playing();
|
|
//!update the stream
|
|
bool update();
|
|
//!checks to make sure everything is ready to go
|
|
bool isvalid();
|
|
|
|
cAudio(IAudioDecoder* decoder);
|
|
~cAudio();
|
|
|
|
protected:
|
|
private:
|
|
//!empties the queue
|
|
void empty();
|
|
//!checks openal error state
|
|
void check();
|
|
//!reloads a buffer
|
|
bool stream(ALuint buffer);
|
|
//!stringify an error code
|
|
std::string errorString(int code);
|
|
|
|
//! front and back buffers
|
|
ALuint buffers[3];
|
|
//! audio source
|
|
ALuint source;
|
|
//! decoder pointer
|
|
IAudioDecoder* Decoder;
|
|
|
|
//! if the file to be played is going to be streamed
|
|
bool streaming;
|
|
//!if the file is to loop
|
|
bool toloop;
|
|
//!if the file should play
|
|
bool playaudio;
|
|
//!if to pause audio
|
|
bool pauseaudio;
|
|
//!if audio is paused
|
|
bool paused();
|
|
};
|
|
}
|
|
#endif //! CAUDIO_H_INCLUDED
|