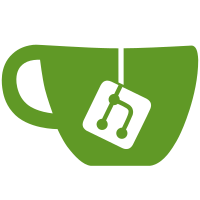
Added the ability for the user to provide a DataSourceFactory, which cAudio will use to get audio data. This interface can be overridden to provide cAudio the ability to read from zip archives, TCP streams, potentially any location. DataSourceFactories can also be prioritized. Made the existing FileSource a factory and registered it by default with the manager. If the user wishes to prevent cAudio from reading from disk, unRegister the "FileSystem" data source. Made tutorials conform with the changes to the API.
84 lines
1.7 KiB
C++
84 lines
1.7 KiB
C++
// Copyright (c) 2008-2010 Raynaldo (Wildicv) Rivera, Joshua (Dark_Kilauea) Jones
|
|
// This file is part of the "cAudio Engine"
|
|
// For conditions of distribution and use, see copyright notice in cAudio.h
|
|
|
|
#include "../Headers/cFileSource.h"
|
|
#include <cstring>
|
|
#include "../Headers/cUtils.h"
|
|
|
|
namespace cAudio
|
|
{
|
|
|
|
//!Init Takes a string for file name
|
|
cFileSource::cFileSource(const char* filename) : pFile(NULL), Valid(false), Filesize(0)
|
|
{
|
|
std::string safeFilename = safeCStr(filename);
|
|
if(safeFilename.length() != 0)
|
|
{
|
|
pFile = fopen(safeFilename.c_str(),"rb");
|
|
if(pFile)
|
|
Valid = true;
|
|
}
|
|
|
|
if(Valid)
|
|
{
|
|
fseek(pFile, 0, SEEK_END);
|
|
Filesize = ftell(pFile);
|
|
fseek(pFile, 0, SEEK_SET);
|
|
}
|
|
}
|
|
|
|
cFileSource::~cFileSource()
|
|
{
|
|
if(pFile)
|
|
fclose(pFile);
|
|
}
|
|
|
|
//!Returns true if the FileSource is valid
|
|
bool cFileSource::isValid()
|
|
{
|
|
return Valid;
|
|
}
|
|
|
|
//!Returns the current position of the file stream.
|
|
int cFileSource::getCurrentPos()
|
|
{
|
|
return ftell(pFile);
|
|
}
|
|
|
|
//!Returns the file size
|
|
int cFileSource::getSize()
|
|
{
|
|
return Filesize;
|
|
}
|
|
|
|
//!Read current FileSource Data
|
|
int cFileSource::read(void* output, int size)
|
|
{
|
|
return fread(output, sizeof(char), size, pFile);
|
|
}
|
|
|
|
//!Seek the file stream
|
|
bool cFileSource::seek(int amount, bool relative)
|
|
{
|
|
if(relative == true)
|
|
{
|
|
int oldamount = ftell(pFile);
|
|
fseek(pFile, amount, SEEK_CUR);
|
|
|
|
//check against the absolute position
|
|
if(oldamount+amount != ftell(pFile))
|
|
return false;
|
|
}
|
|
else
|
|
{
|
|
fseek(pFile, amount, SEEK_SET);
|
|
if(amount != ftell(pFile))
|
|
return false;
|
|
}
|
|
|
|
return true;
|
|
}
|
|
|
|
};
|