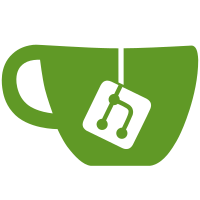
Made cAudio, cAudioCapture, cAudioManager and other classes thread safe. Made cAudioManager use a seperate update thread by default. Added cAudioSleep, a cross-platform sleep() function Moved global defines to cAudioDefines Added defines to disable thread safety or the internal update thread if the user wishes it Updated tutorials to reflect the changes made
111 lines
3.4 KiB
C++
111 lines
3.4 KiB
C++
#include <iostream>
|
|
#include <string>
|
|
//Include IAudioManager so we can easily work with cAudio
|
|
#include "../../include/IAudioManager.h"
|
|
//Include IAudio so we can create cAudio objects
|
|
#include "../../include/IAudio.h"
|
|
//Include our version of Sleep to free CPU usage
|
|
#include "../../include/cAudioSleep.h"
|
|
|
|
using namespace std;
|
|
|
|
#define CAPTURE_FREQUENCY 22050
|
|
#define CAPTURE_DURATION 10
|
|
#define CAPTURE_FORMAT cAudio::EAF_16BIT_MONO
|
|
|
|
int main(int argc, char* argv[])
|
|
{
|
|
//To make visual studio happy
|
|
cAudio::IAudio* mysound = NULL;
|
|
|
|
//Some fancy text
|
|
cout << "cAudio 1.7.1 Tutorial 4: Capturing Audio \n \n";
|
|
|
|
std::string formatName;
|
|
|
|
if(CAPTURE_FORMAT == cAudio::EAF_8BIT_MONO)
|
|
formatName = "8 Bit Mono";
|
|
else if(CAPTURE_FORMAT == cAudio::EAF_8BIT_STEREO)
|
|
formatName = "8 Bit Stereo";
|
|
else if(CAPTURE_FORMAT == cAudio::EAF_16BIT_MONO)
|
|
formatName = "16 Bit Mono";
|
|
else
|
|
formatName = "16 Bit Stereo";
|
|
|
|
//Grap the cAudioManager
|
|
cAudio::IAudioManager* manager = cAudio::getAudioManager();
|
|
//Init the cAudio Engine
|
|
manager->init(argc,argv);
|
|
|
|
//! The capture interface should be grabbed after the manager has been initialized
|
|
cAudio::IAudioCapture* capture = manager->getAudioCapture();
|
|
bool captureReady = false;
|
|
cout << "Capturing Supported: " << std::boolalpha << capture->isSupported() << "\n";
|
|
if(capture->isSupported())
|
|
{
|
|
captureReady = capture->initialize(CAPTURE_FREQUENCY, CAPTURE_FORMAT);
|
|
cout << "Ready to capture audio: " << std::boolalpha << captureReady << "\n \n";
|
|
|
|
//Quick math to figure out how large our data should be for the duration of the record time
|
|
const int targetRecordSize = CAPTURE_FREQUENCY * CAPTURE_DURATION * capture->getSampleSize();
|
|
cout << "Capture Frequency: " << CAPTURE_FREQUENCY << "\n";
|
|
cout << "Capture Duration: " << CAPTURE_DURATION << "\n";
|
|
cout << "Capture Format: " << formatName << "\n";
|
|
cout << "Sample Size: " << capture->getSampleSize() << "\n";
|
|
cout << "Total size of audio: " << targetRecordSize << "\n";
|
|
|
|
cout << std::endl;
|
|
|
|
int currentsize = 0;
|
|
cout << "Starting capture... \n";
|
|
if(capture->beginCapture())
|
|
{
|
|
while(currentsize < targetRecordSize)
|
|
{
|
|
currentsize = capture->getCurrentCapturedAudioSize();
|
|
|
|
//Sleep for 1 ms to free some CPU
|
|
cAudio::cAudioSleep(1);
|
|
}
|
|
}
|
|
capture->stopCapture();
|
|
cout << "Capture stopped... \n \n";
|
|
|
|
//Grab the total size again, ensures we get ALL the audio data
|
|
//Not completely necessary, as starting a capture again will clear the old audio data
|
|
currentsize = capture->getCurrentCapturedAudioSize();
|
|
|
|
char* buffer = new char[currentsize];
|
|
capture->getCapturedAudio(buffer, currentsize);
|
|
|
|
//Create a IAudio object and load a sound from a file
|
|
mysound = manager->createFromRaw("sound1", buffer, currentsize, CAPTURE_FREQUENCY, CAPTURE_FORMAT);
|
|
|
|
delete buffer;
|
|
|
|
if(mysound)
|
|
{
|
|
cout << "Playing back captured audio... \n \n";
|
|
mysound->setVolume(1.0);
|
|
//Set the IAudio Sound to play2d and loop
|
|
mysound->play2d(false);
|
|
|
|
while(mysound->playing())
|
|
{
|
|
//Sleep for 10 ms to free some CPU
|
|
cAudio::cAudioSleep(10);
|
|
}
|
|
}
|
|
}
|
|
|
|
//Delete all IAudio sounds
|
|
manager->release();
|
|
//Shutdown cAudio
|
|
manager->shutDown();
|
|
|
|
std::cout << "Press any key to quit \n";
|
|
std::cin.get();
|
|
|
|
return 0;
|
|
}
|