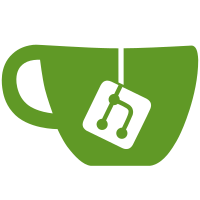
Allow for settings to be described by something other than a DHCP option tag if desirable. Currently used only for the MAC address setting. Separate out fake DHCP packet creation code from dhcp.c to fakedhcp.c. Remove notion of settings from dhcppkt.c. Rationalise dhcp.c to use settings API only for final registration of the DHCP options, rather than using {store,fetch}_setting throughout.
91 lines
2.7 KiB
C
91 lines
2.7 KiB
C
/*
|
|
* Copyright (C) 2008 Michael Brown <mbrown@fensystems.co.uk>.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation; either version 2 of the
|
|
* License, or any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful, but
|
|
* WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 675 Mass Ave, Cambridge, MA 02139, USA.
|
|
*/
|
|
|
|
#include <string.h>
|
|
#include <errno.h>
|
|
#include <gpxe/dhcp.h>
|
|
#include <gpxe/settings.h>
|
|
#include <gpxe/netdevice.h>
|
|
|
|
/** @file
|
|
*
|
|
* Network device configuration settings
|
|
*
|
|
*/
|
|
|
|
/** Network device named settings */
|
|
struct setting mac_setting __setting = {
|
|
.name = "mac",
|
|
.description = "MAC address",
|
|
.type = &setting_type_hex,
|
|
};
|
|
|
|
/**
|
|
* Store value of network device setting
|
|
*
|
|
* @v settings Settings block
|
|
* @v setting Setting to store
|
|
* @v data Setting data, or NULL to clear setting
|
|
* @v len Length of setting data
|
|
* @ret rc Return status code
|
|
*/
|
|
static int netdev_store ( struct settings *settings, struct setting *setting,
|
|
const void *data, size_t len ) {
|
|
struct net_device *netdev = container_of ( settings, struct net_device,
|
|
settings.settings );
|
|
|
|
if ( setting_cmp ( setting, &mac_setting ) == 0 ) {
|
|
if ( len != netdev->ll_protocol->ll_addr_len )
|
|
return -EINVAL;
|
|
memcpy ( netdev->ll_addr, data, len );
|
|
return 0;
|
|
} else {
|
|
return simple_settings_store ( settings, setting, data, len );
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Fetch value of network device setting
|
|
*
|
|
* @v settings Settings block
|
|
* @v setting Setting to fetch
|
|
* @v data Setting data, or NULL to clear setting
|
|
* @v len Length of setting data
|
|
* @ret rc Return status code
|
|
*/
|
|
static int netdev_fetch ( struct settings *settings, struct setting *setting,
|
|
void *data, size_t len ) {
|
|
struct net_device *netdev = container_of ( settings, struct net_device,
|
|
settings.settings );
|
|
|
|
if ( setting_cmp ( setting, &mac_setting ) == 0 ) {
|
|
if ( len > netdev->ll_protocol->ll_addr_len )
|
|
len = netdev->ll_protocol->ll_addr_len;
|
|
memcpy ( data, netdev->ll_addr, len );
|
|
return netdev->ll_protocol->ll_addr_len;
|
|
} else {
|
|
return simple_settings_fetch ( settings, setting, data, len );
|
|
}
|
|
}
|
|
|
|
/** Network device configuration settings operations */
|
|
struct settings_operations netdev_settings_operations = {
|
|
.store = netdev_store,
|
|
.fetch = netdev_fetch,
|
|
};
|